一、numpy概述
numpy(Numerical Python)提供了python對多維數組對象的支持:ndarray,具有矢量運算能力,快速、節省空間。numpy支持高級大量的維度數組與矩陣運算,此外也針對數組運算提供大量的數學函數庫。
二、創建ndarray數組
ndarray:N維數組對象(矩陣),所有元素必須是相同類型。ndarray屬性:ndim屬性,表示維度個數;shape屬性,表示各維度大??;dtype屬性,表示數據類型。
創建ndarray數組函數:
代碼示例:
# -*- coding: utf-8 -*- import numpy; print '使用列表生成一維數組' data = [1,2,3,4,5,6] x = numpy.array(data) print x #打印數組 print x.dtype #打印數組元素的類型 print '使用列表生成二維數組' data = [[1,2],[3,4],[5,6]] x = numpy.array(data) print x #打印數組 print x.ndim #打印數組的維度 print x.shape #打印數組各個維度的長度。shape是一個元組 print '使用zero/ones/empty創建數組:根據shape來創建' x = numpy.zeros(6) #創建一維長度為6的,元素都是0一維數組 print x x = numpy.zeros((2,3)) #創建一維長度為2,二維長度為3的二維0數組 print x x = numpy.ones((2,3)) #創建一維長度為2,二維長度為3的二維1數組 print x x = numpy.empty((3,3)) #創建一維長度為2,二維長度為3,未初始化的二維數組 print x print '使用arrange生成連續元素' print numpy.arange(6) # [0,1,2,3,4,5,] 開區間 print numpy.arange(0,6,2) # [0, 2,4]
三、指定ndarray數組元素的類型
NumPy數據類型:
代碼示例:
print '生成指定元素類型的數組:設置dtype屬性' x = numpy.array([1,2.6,3],dtype = numpy.int64) print x # 元素類型為int64 print x.dtype x = numpy.array([1,2,3],dtype = numpy.float64) print x # 元素類型為float64 print x.dtype print '使用astype復制數組,并轉換類型' x = numpy.array([1,2.6,3],dtype = numpy.float64) y = x.astype(numpy.int32) print y # [1 2 3] print x # [ 1. 2.6 3. ] z = y.astype(numpy.float64) print z # [ 1. 2. 3.] print '將字符串元素轉換為數值元素' x = numpy.array(['1','2','3'],dtype = numpy.string_) y = x.astype(numpy.int32) print x # ['1' '2' '3'] print y # [1 2 3] 若轉換失敗會拋出異常 print '使用其他數組的數據類型作為參數' x = numpy.array([ 1., 2.6,3. ],dtype = numpy.float32); y = numpy.arange(3,dtype=numpy.int32); print y # [0 1 2] print y.astype(x.dtype) # [ 0. 1. 2.]
四、ndarray的矢量化計算
矢量運算:相同大小的數組鍵間的運算應用在元素上矢量和標量運算:“廣播”— 將標量“廣播”到各個元素
代碼示例:
print 'ndarray數組與標量/數組的運算' x = numpy.array([1,2,3]) print x*2 # [2 4 6] print x>2 # [False False True] y = numpy.array([3,4,5]) print x+y # [4 6 8] print x>y # [False False False]
五、ndarray數組的基本索引和切片
一維數組的索引:與Python的列表索引功能相似
多維數組的索引:
arr[r1:r2, c1:c2]
arr[1,1] 等價 arr[1][1]
[:] 代表某個維度的數據
代碼示例:
print 'ndarray的基本索引' x = numpy.array([[1,2],[3,4],[5,6]]) print x[0] # [1,2] print x[0][1] # 2,普通python數組的索引 print x[0,1] # 同x[0][1],ndarray數組的索引 x = numpy.array([[[1, 2], [3,4]], [[5, 6], [7,8]]]) print x[0] # [[1 2],[3 4]] y = x[0].copy() # 生成一個副本 z = x[0] # 未生成一個副本 print y # [[1 2],[3 4]] print y[0,0] # 1 y[0,0] = 0 z[0,0] = -1 print y # [[0 2],[3 4]] print x[0] # [[-1 2],[3 4]] print z # [[-1 2],[3 4]] print 'ndarray的切片' x = numpy.array([1,2,3,4,5]) print x[1:3] # [2,3] 右邊開區間 print x[:3] # [1,2,3] 左邊默認為 0 print x[1:] # [2,3,4,5] 右邊默認為元素個數 print x[0:4:2] # [1,3] 下標遞增2 x = numpy.array([[1,2],[3,4],[5,6]]) print x[:2] # [[1 2],[3 4]] print x[:2,:1] # [[1],[3]] x[:2,:1] = 0 # 用標量賦值 print x # [[0,2],[0,4],[5,6]] x[:2,:1] = [[8],[6]] # 用數組賦值 print x # [[8,2],[6,4],[5,6]]
六、ndarray數組的布爾索引和花式索引
布爾索引:使用布爾數組作為索引。arr[condition],condition為一個條件/多個條件組成的布爾數組。
布爾型索引代碼示例:
print 'ndarray的布爾型索引' x = numpy.array([3,2,3,1,3,0]) # 布爾型數組的長度必須跟被索引的軸長度一致 y = numpy.array([True,False,True,False,True,False]) print x[y] # [3,3,3] print x[y==False] # [2,1,0] print x>=3 # [ True False True False True False] print x[~(x>=3)] # [2,1,0] print (x==2)|(x==1) # [False True False True False False] print x[(x==2)|(x==1)] # [2 1] x[(x==2)|(x==1)] = 0 print x # [3 0 3 0 3 0]
花式索引:使用整型數組作為索引。
花式索引代碼示例:
print 'ndarray的花式索引:使用整型數組作為索引' x = numpy.array([1,2,3,4,5,6]) print x[[0,1,2]] # [1 2 3] print x[[-1,-2,-3]] # [6,5,4] x = numpy.array([[1,2],[3,4],[5,6]]) print x[[0,1]] # [[1,2],[3,4]] print x[[0,1],[0,1]] # [1,4] 打印x[0][0]和x[1][1] print x[[0,1]][:,[0,1]] # 打印01行的01列 [[1,2],[3,4]] # 使用numpy.ix_()函數增強可讀性 print x[numpy.ix_([0,1],[0,1])] #同上 打印01行的01列 [[1,2],[3,4]] x[[0,1],[0,1]] = [0,0] print x # [[0,2],[3,0],[5,6]]
七、ndarray數組的轉置和軸對換
數組的轉置/軸對換只會返回源數據的一個視圖,不會對源數據進行修改。
代碼示例:
print 'ndarray數組的轉置和軸對換' k = numpy.arange(9) #[0,1,....8] m = k.reshape((3,3)) # 改變數組的shape復制生成2維的,每個維度長度為3的數組 print k # [0 1 2 3 4 5 6 7 8] print m # [[0 1 2] [3 4 5] [6 7 8]] # 轉置(矩陣)數組:T屬性 : mT[x][y] = m[y][x] print m.T # [[0 3 6] [1 4 7] [2 5 8]] # 計算矩陣的內積 xTx print numpy.dot(m,m.T) # numpy.dot點乘 # 高維數組的軸對象 k = numpy.arange(8).reshape(2,2,2) print k # [[[0 1],[2 3]],[[4 5],[6 7]]] print k[1][0][0] # 軸變換 transpose 參數:由軸編號組成的元組 m = k.transpose((1,0,2)) # m[y][x][z] = k[x][y][z] print m # [[[0 1],[4 5]],[[2 3],[6 7]]] print m[0][1][0] # 軸交換 swapaxes (axes:軸),參數:一對軸編號 m = k.swapaxes(0,1) # 將第一個軸和第二個軸交換 m[y][x][z] = k[x][y][z] print m # [[[0 1],[4 5]],[[2 3],[6 7]]] print m[0][1][0] # 使用軸交換進行數組矩陣轉置 m = numpy.arange(9).reshape((3,3)) print m # [[0 1 2] [3 4 5] [6 7 8]] print m.swapaxes(1,0) # [[0 3 6] [1 4 7] [2 5 8]]
八、ndarray通用函數
通用函數(ufunc)是一種對ndarray中的數據執行元素級運算的函數。
一元ufunc:
一元ufunc代碼示例:
print '一元ufunc示例' x = numpy.arange(6) print x # [0 1 2 3 4 5] print numpy.square(x) # [ 0 1 4 9 16 25] x = numpy.array([1.5,1.6,1.7,1.8]) y,z = numpy.modf(x) print y # [ 0.5 0.6 0.7 0.8] print z # [ 1. 1. 1. 1.]
二元ufunc:
二元ufunc代碼示例:
print '二元ufunc示例' x = numpy.array([[1,4],[6,7]]) y = numpy.array([[2,3],[5,8]]) print numpy.maximum(x,y) # [[2,4],[6,8]] print numpy.minimum(x,y) # [[1,3],[5,7]]
九、NumPy的where函數使用
np.where(condition, x, y),第一個參數為一個布爾數組,第二個參數和第三個參數可以是標量也可以是數組。
代碼示例:
print 'where函數的使用' cond = numpy.array([True,False,True,False]) x = numpy.where(cond,-2,2) print x # [-2 2 -2 2] cond = numpy.array([1,2,3,4]) x = numpy.where(cond>2,-2,2) print x # [ 2 2 -2 -2] y1 = numpy.array([-1,-2,-3,-4]) y2 = numpy.array([1,2,3,4]) x = numpy.where(cond>2,y1,y2) # 長度須匹配 print x # [1,2,-3,-4] print 'where函數的嵌套使用' y1 = numpy.array([-1,-2,-3,-4,-5,-6]) y2 = numpy.array([1,2,3,4,5,6]) y3 = numpy.zeros(6) cond = numpy.array([1,2,3,4,5,6]) x = numpy.where(cond>5,y3,numpy.where(cond>2,y1,y2)) print x # [ 1. 2. -3. -4. -5. 0.]
十、ndarray常用的統計方法
可以通過這些基本統計方法對整個數組/某個軸的數據進行統計計算。
代碼示例:
print 'numpy的基本統計方法' x = numpy.array([[1,2],[3,3],[1,2]]) #同一維度上的數組長度須一致 print x.mean() # 2 print x.mean(axis=1) # 對每一行的元素求平均 print x.mean(axis=0) # 對每一列的元素求平均 print x.sum() #同理 12 print x.sum(axis=1) # [3 6 3] print x.max() # 3 print x.max(axis=1) # [2 3 2] print x.cumsum() # [ 1 3 6 9 10 12] print x.cumprod() # [ 1 2 6 18 18 36]
用于布爾數組的統計方法:
sum : 統計數組/數組某一維度中的True的個數
any: 統計數組/數組某一維度中是否存在一個/多個True
all:統計數組/數組某一維度中是否都是True
代碼示例:
print '用于布爾數組的統計方法' x = numpy.array([[True,False],[True,False]]) print x.sum() # 2 print x.sum(axis=1) # [1,1] print x.any(axis=0) # [True,False] print x.all(axis=1) # [False,False]
使用sort對數組/數組某一維度進行就地排序(會修改數組本身)。
代碼示例:
print '.sort的就地排序' x = numpy.array([[1,6,2],[6,1,3],[1,5,2]]) x.sort(axis=1) print x # [[1 2 6] [1 3 6] [1 2 5]] #非就地排序:numpy.sort()可產生數組的副本
十一、ndarray數組的去重以及集合運算
代碼示例:(方法返回類型為一維數組(1d))
print 'ndarray的唯一化和集合運算' x = numpy.array([[1,6,2],[6,1,3],[1,5,2]]) print numpy.unique(x) # [1,2,3,5,6] y = numpy.array([1,6,5]) print numpy.in1d(x,y) # [ True True False True True False True True False] print numpy.setdiff1d(x,y) # [2 3] print numpy.intersect1d(x,y) # [1 5 6]
十二、numpy中的線性代數
import numpy.linalg 模塊。線性代數(linear algebra)
常用的numpy.linalg模塊函數:
代碼示例:
print '線性代數' import numpy.linalg as nla print '矩陣點乘' x = numpy.array([[1,2],[3,4]]) y = numpy.array([[1,3],[2,4]]) print x.dot(y) # [[ 5 11][11 25]] print numpy.dot(x,y) # # [[ 5 11][11 25]] print '矩陣求逆' x = numpy.array([[1,1],[1,2]]) y = nla.inv(x) # 矩陣求逆(若矩陣的逆存在) print x.dot(y) # 單位矩陣 [[ 1. 0.][ 0. 1.]] print nla.det(x) # 求行列式
十三、numpy中的隨機數生成
import numpy.random模塊。
常用的numpy.random模塊函數:
代碼示例:
print 'numpy.random隨機數生成' import numpy.random as npr x = npr.randint(0,2,size=100000) #拋硬幣 print (x>0).sum() # 正面的結果 print npr.normal(size=(2,2)) #正態分布隨機數數組 shape = (2,2)
十四、ndarray數組重塑
代碼示例:
print 'ndarray數組重塑' x = numpy.arange(0,6) #[0 1 2 3 4] print x #[0 1 2 3 4] print x.reshape((2,3)) # [[0 1 2][3 4 5]] print x #[0 1 2 3 4] print x.reshape((2,3)).reshape((3,2)) # [[0 1][2 3][4 5]] y = numpy.array([[1,1,1],[1,1,1]]) x = x.reshape(y.shape) print x # [[0 1 2][3 4 5]] print x.flatten() # [0 1 2 3 4 5] x.flatten()[0] = -1 # flatten返回的是拷貝 print x # [[0 1 2][3 4 5]] print x.ravel() # [0 1 2 3 4 5] x.ravel()[0] = -1 # ravel返回的是視圖(引用) print x # [[-1 1 2][3 4 5]] print "維度大小自動推導" arr = numpy.arange(15) print arr.reshape((5, -1)) # 15 / 5 = 3
十五、ndarray數組的拆分與合并
代碼示例:
print '數組的合并與拆分' x = numpy.array([[1, 2, 3], [4, 5, 6]]) y = numpy.array([[7, 8, 9], [10, 11, 12]]) print numpy.concatenate([x, y], axis = 0) # 豎直組合 [[ 1 2 3][ 4 5 6][ 7 8 9][10 11 12]] print numpy.concatenate([x, y], axis = 1) # 水平組合 [[ 1 2 3 7 8 9][ 4 5 6 10 11 12]] print '垂直stack與水平stack' print numpy.vstack((x, y)) # 垂直堆疊:相對于垂直組合 print numpy.hstack((x, y)) # 水平堆疊:相對于水平組合 # dstack:按深度堆疊 print numpy.split(x,2,axis=0) # 按行分割 [array([[1, 2, 3]]), array([[4, 5, 6]])] print numpy.split(x,3,axis=1) # 按列分割 [array([[1],[4]]), array([[2],[5]]), array([[3],[6]])] # 堆疊輔助類 import numpy as np arr = np.arange(6) arr1 = arr.reshape((3, 2)) arr2 = np.random.randn(3, 2) print 'r_用于按行堆疊' print np.r_[arr1, arr2] ''' [[ 0. 1. ] [ 2. 3. ] [ 4. 5. ] [ 0.22621904 0.39719794] [-1.2201912 -0.23623549] [-0.83229114 -0.72678578]] ''' print 'c_用于按列堆疊' print np.c_[np.r_[arr1, arr2], arr] ''' [[ 0. 1. 0. ] [ 2. 3. 1. ] [ 4. 5. 2. ] [ 0.22621904 0.39719794 3. ] [-1.2201912 -0.23623549 4. ] [-0.83229114 -0.72678578 5. ]] ''' print '切片直接轉為數組' print np.c_[1:6, -10:-5] ''' [[ 1 -10] [ 2 -9] [ 3 -8] [ 4 -7] [ 5 -6]] '''
十六、數組的元素重復操作
代碼示例:
print '數組的元素重復操作' x = numpy.array([[1,2],[3,4]]) print x.repeat(2) # 按元素重復 [1 1 2 2 3 3 4 4] print x.repeat(2,axis=0) # 按行重復 [[1 2][1 2][3 4][3 4]] print x.repeat(2,axis=1) # 按列重復 [[1 1 2 2][3 3 4 4]] x = numpy.array([1,2]) print numpy.tile(x,2) # tile瓦片:[1 2 1 2] print numpy.tile(x, (2, 2)) # 指定從低維到高維依次復制的次數。 # [[1 2 1 2][1 2 1 2]]
-
函數
+關注
關注
3文章
4117瀏覽量
61507 -
python
+關注
關注
52文章
4700瀏覽量
83647 -
數組
+關注
關注
1文章
409瀏覽量
25675
原文標題:python之numpy的基本使用
文章出處:【微信號:gh_0d472ef09794,微信公眾號:Zero機器學習與人工智能】歡迎添加關注!文章轉載請注明出處。
發布評論請先 登錄
相關推薦
什么是NumPy?選擇NUMPY的原因及其工作原理是什么
python 學習:在內網中 python庫-numpy 安裝方法,升級pip3版本的指令
有關Python的解析
Python中NumPy擴展包簡介及案例詳解
Python的兩個基礎包numpy和Matplotlib示例詳解
靈活運用Python中numpy庫的矩陣運算
數據分析必備的NumPy技巧(Python)
用于Python的英特爾,加速NUMPY和SCIPY技術
用于數據科學的python必學模塊之Numpy的備忘單資料免費下載
如何使用Python和Numpy等技術實現圖像處理
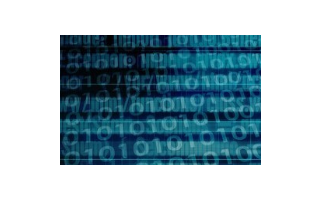
詳解Python中的Pandas和Numpy庫
Python編程語言開源庫NUMPY的工作原理及優勢
List和Numpy Array有什么區別
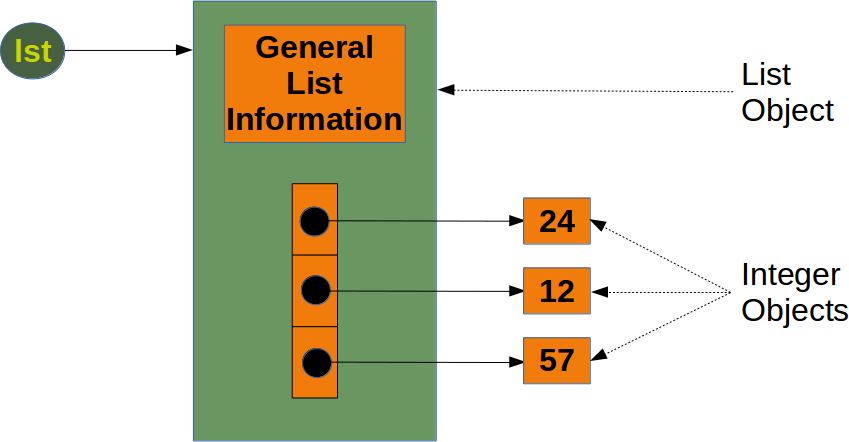
評論